In this post, I will discuss about Unformatted Console I/O Functions, Using gets( ) and puts( ) in C programming, File Operations: A program to display content of file, Opening a File using function in C, File handling and File allocation concepts and Reading from a File in C programming language.
Unformatted Console I/O Functions:
Unformatted console I/O are the function which can handle single character and also can handle string of character and are plenty in number. We have used most of the time scanf() which has a limitation then until the Enter key is hit before function can take the input. However we can use getch() and getche() are that can used for reading single character the instant it is typed without waiting to hit Enter key. These functions return the character that has been most recently typed. The ‘e’ in getche( ) function means it shows the character that you typed to the screen. As against this getch( ) just returns the character that is typed without showing it on the screen. Function getchar( ) works similarly and displays the character that is typed on the screen, but requires Enter key to be typed following the character that is typed. The difference between getchar( ) and fgetchar( ) is that former is a macro whereas the latter is a function.
Header file for getch( ) and getche( )is conio.h. The macro getchar( ) and header file for fgetchar( )are stdio.h. Below mentioned is a program explaining about unformatted console I/O function.
#include<stdio.h>
#include<conio.h>
void main( )
{
char ch ;
printf ( “\nYou have Entered More Journals” ) ;
getch( ) ; /* will not echo the character */
printf ( “\nMore Journals is Technology site” ) ;
ch = getche( ) ; /* will echo the character typed */
printf ( “\nMore Journals is the best” ) ;
getchar( ) ; /* will echo character, must be followed by enter key */
printf ( “\nContinue Y/N” ) ;
fgetchar( ) ; /* will echo character, must be followed by enter key */
}
Using gets( ) and puts( ) in C programming:
Function gets() receives a string from keyboard because scanf() function has few limitation while receiving string of character. Below mentioned is an example:
#include<stdio.h>
void main( )
{
char name[50] ;
printf ( “\nEnter name of Blog: ” ) ;
scanf ( “%s”, name ) ;
printf ( “%s”, name ) ;
}
Output of above program is:
Enter name of Blog: More Journals
File Operations: A program to display content of file
/* Display contents of a file on screen. */
# include <stdio.h>
void main( )
{
FILE *fp ;
char ch ;
fp = fopen ( “PR1.C”, “r” ) ;
while ( 1 )
{
ch = fgetc ( fp ) ;
if ( ch == EOF )
break ;
printf ( “%c”, ch ) ;
}
fclose ( fp ) ;
}
Opening a File using function in C:
To performing reading and writing information from a file on disk first we need to know how to open the file. To open the file we call a function fopen(). This function opens the mentioned file in read mode. Compiler thinks the we will be only reading the content of the file. Function fopen() performs three important tasks when a file is opened in “r” mode:
(a) Firstly it searches on the disk the file to be opened.
(b) Then it loads the file from the disk into a place in memory called buffer.
(c) It sets up a character pointer that points to the first character of the buffer.
File handling and File allocation concepts:
All the operation like reading a file, information like mode of opening, size of file, place in the file from where the next read operation would be performed has to be maintained. Since every information is inter related, therefore all the information is gathered by fopen() in a structure called FILE.fopen() returns the address of which is collected in the structure pointer called fp. We can declare fp as
FILE *fp ;
File structure is defined in stdio.h header file.
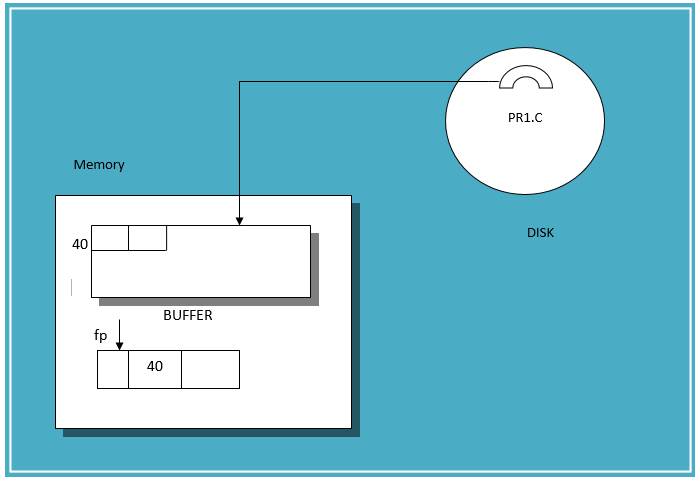
Reading from a File:
Once the file is opened for reading using fopen(), partial or full files content will be brought to buffer and a pointer is set up that points to first character in the buffer.
To read the file’s contents from memory there exists a function called fgetc(). This has been used in program as:
ch = fgetc ( fp ) ;
fgetc() reads the characters from current pointer position and advance the pointer position so that it points to the next character and returns the character to read and which is collected in variable ch. Once the file is opened it will be no longer referred by file name instead with fp.
We have used the function fgetc() with indefinite while loop and will be coming out when it reaches the end of file. End of file is arrived when a special character with ASCII value is 26. This character is inserted beyond the last character in file.
While reading from the file, when fgetc( ) encounters this special character, instead of returning the character that it has read, it returns the macro EOF. The EOF macro has been defined in the file “stdio.h”. In place of the function fgetc( ) we could have as well used the macro getc( ) with the same effect.