In this post, I will discuss about Important Points for Declaring a Structure, How Structure Elements are Stored, Array of Structures, Types of Input / Output, Console Input-Output Functions, Formatted Console Input / Output Functions, Format Specification, Optional Format Specifiers, and Escape Sequences in C language.
Important Points for Declaring a Structure:
Below mentioned are the points to be considered while declaring a structure:
(a) Semicolon should be present after closing braces in structure type declaration.
(b)Structure type declaration will no tell the compiler to reserve any space in memory. It tells only the form of structure.
(c)Structure type declaration will be shown at the top of source code file, even before any variables or function are defined. Structure will be available in separate header file and while is included whichever program will requiring the defined structure.
(d) In structure if a variable is initialized to a value, then all the all the elements are set to value. This is quite easy for initializing structure variables. If we will not be doing this way then, we will have to initialize each elements to zero.
How Structure Elements are Stored:
Elements of structure are always stored in successive memory location. A program is written below for explanation:
/*A program to memory storing of structure elements*/
#include<stdio.h>
void main( )
{
struct emp
{
char name ;
float salary ;
int age ;
} ;
struct emp e1 = { ‘Steve’, 130.00, 30 } ;
printf ( “\nAddress of Name = %u”, &e1.name ) ;
printf ( “\nAddress of Salary = %u”, eb1.salary ) ;
printf ( “\nAddress of Age = %u”, &e1.age ) ;
}
Output of the above mentioned program is :
Address of Name = 65518
Address of Salary = 65519
Address of Age = 65520
Actually the structure elements are stored in memory as shown in the Figure below:
e1.name e1.salary e1age
ʽSteveʼ 130.00 30
65518 65519 65520
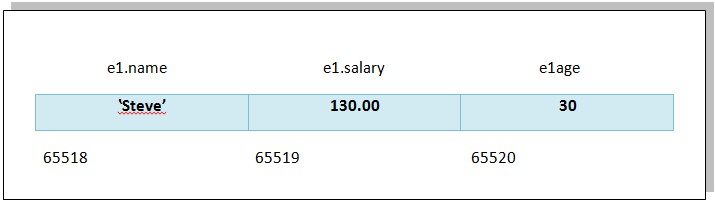
Array of Structures:
For creating 50 different structure if we will creating one by one then it will be very tedious to do that therefore we will be using array of structures. A program is written below which explain how array of structure are written:
/* A program for usage of an array of structures */
#include<stdio.h>
void main( )
{
struct emp
{
char name ;
float salary ;
int age ;
} ;
struct emp b[50] ;
int i ;
for ( i = 1 ; i <= 50 ; i++ )
{
printf ( “\nEnter name, salary and age of employees” ) ;
scanf ( “%c %f %d”, &e[i].name, &e[i].salary, &e[i].age ) ;
}
for ( i = 1 ; i <= 50 ; i++ )
printf ( “\n%c %f %d”, e[i].name, e[i].salary, e[i].age ) ;
}
linkfloat( )
{
float a = 0, *b ;
b = &a ; /* cause emulator to be linked */
a = *b ; /* suppress the warning – variable not used */
}
}
Types of Input / Output:
Every operating system has its own facility for inputting and outputting data, files. Small programs to be written that would link the C compiler for particular operating system.
There are several standard I/O functions and are kept in libraries. These libraries are available with all C compilers.
Different operating systems will having different way of showing output.
For example: the standard library function printf( ) for DOS based C compiler has been written in such a way DOS outputs characters to screen. Similarly, the printf( ) function for a Unixbased compiler has been in a way Unix outputs characters to screen. When we are writing program we don’t have to bother about how the different standard library functions are written and how is functions.
Library functions available for input output are too many. Library files can be classified in to two broad categories.
a) Console I / O Functions Functions to receive input from keyboard and write output to VDU.
b) File I / O Functions Functions to perform I / O operations on a floppy disk or hard disk.
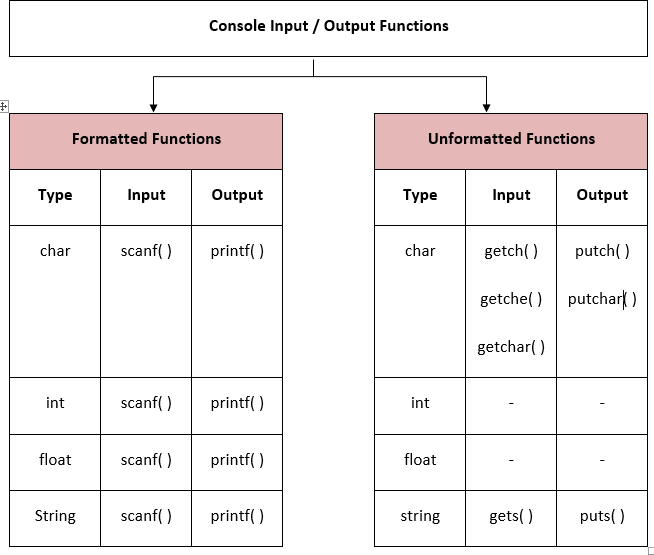
Console Input-Output Functions:
Screen and keyboard are called as Console. Console I/O functions can be classified into two categories – Formatted and Unformatted console I/O functions. Difference between the formatted and unformatted functions is that for formatted functions allows the input read from the keyboard or the output displayed on the VDU to be formatted as per requirement.
For example, if values of average salary and percentage marks are to be displayed on the screen, then the details like where this output would appear on the screen, how many spaces would be present between the two values, the number of places after the decimal points, etc. can be controlled using formatted functions. The functions available under each of these two categories are shown in figure below.
Formatted Console Input / Output Functions:
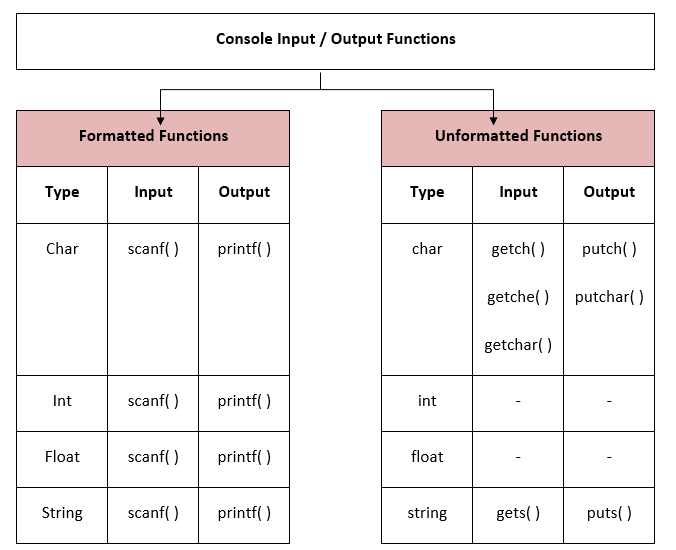
As mentioned above printf( ), and scanf( ) are formatted console I/O functions type. Print and Scanf are functions that allows us to support the provide input in a fixed format. An example for printf is mentioned below:
printf ( “format string”, list of variables ) ;
The format string can contain:
(a) Characters that are simply printed as they are.
(b) Conversion specifications that begin with a % sign
(c) Escape sequences that begin with a \ sign
Below mentioned is a program written:
#include<stdio.h>
void main( )
{
int avg = 150 ;
float per = 79.2 ;
printf ( “Average = %d\nPercentage = %f”, avg, per ) ;
}
The output of the program would be…
Average = 150
Percentage = 79.200000
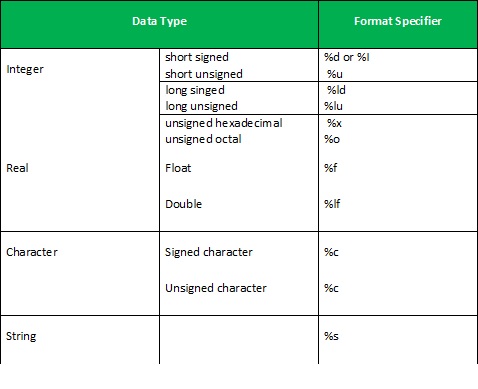
Format Specification:
The %d and %f used in the printf( ) function are called as format specifiers. They tell printf( ) to print the value of avg as a decimal integer and the value of per as a float. Following is the list of format specifiers that can be used with printf( ) function.
Data Type Format Specifier
Integer short signed %d or %I
short unsigned %u
long singed %ld
long unsigned %lu
unsigned hexadecimal %x
unsigned octal %o
Real Float
Double %f
%lf
Character Signed character
Unsigned character %c
%c
String %s
Optional Format Specifiers:
We can provide following optional specifies in the format specifications.
Specifier Description
dd
. Digits specifying field width.
Decimal point separating field width from precision (precision stands for the number of places after the decimal point)
dd
– Digits specifying precision.
Minus sign for left justifying the output in the specified field width.
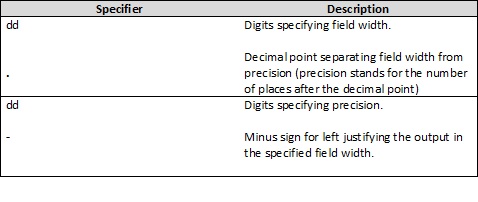
Escape Sequences:
Newline character if inserted in printf() then output will be from the beginning of next line. Newline character is an escape sequence because ‘\’ is considered as escape character.
Below mentioned example displays the usage of ‘\n’ and new escape sequence \t. \t moves the cursor to next tab.
#include<stdio.h>
void main( )
{
printf ( “Best\tblog \tis \nthats\ttechnology” ) ;
}
Best blog is
thats technology
The \n character causes a new line to begin following ‘is’. The tab and newline are probably the most commonly used escape sequences, but there are others as well. Below mentioned is list of escape sequences:
Escape Sequence Purpose Escape Sequence Purpose
\n New Line \t Tab
\b Backspace \r Carriage Return
\f Form Feed \a Alert
\’ Single Quote \” Double Quote
\\ Backslash
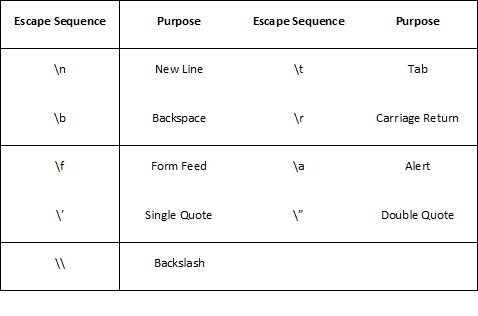