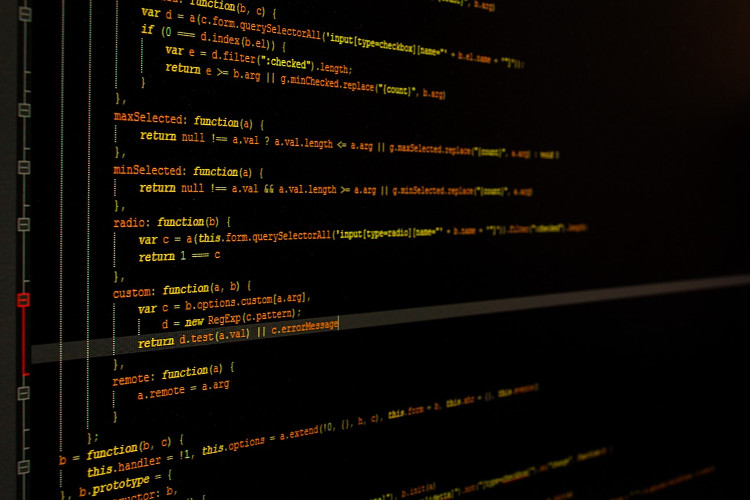
In this post, I will discuss about strings, Program to Demonstrate Printing of a String, %s Format Specifier, scanf( ), Standard Library String Functions in C, strlen( ) function, strcpy( ) function, Function to concatenate a string, Function of comparison of two stings in C, Structure (Grouping of data type together in C), and Declaring a Structure in C programming.
What are Strings?
Strings are group of characters which can be stored in character array, just like group of integers can be stored in integer array. Strings are treated by as character arrays in many languages. Strings are used in programs to get desired output from text.
A string is one dimensional array terminated by null (‘\0’).
Char name [] = {‘M’, ‘O’, ‘R’, ‘E’, ‘J’, ‘O’, ‘U’, ‘R’, ‘N’, ‘A’, ‘L’, ‘S’, ‘\0’}
Last character in a string will always be ‘\0’ and each character will be taking one byte of memory. ‘\0’ is called as null characters and ASCII value is 0/ Null character is not equivalent to 0. Below mentioned figure explains about the memory allocation. Characters of array will be stored in contiguous location. Having a null character at the end of the array helps the function to determine where the string or a collection of characters ends.
Strings will be very often used therefore below mentioned is an example from where initializing a string will be easier while initializing we don’t need to enter null character because C will be doing it automatically.
Char x [] = ”MORE JOURNALS” ;
Program to Demonstrate Printing of a String:
#include<stdio.h>
void main( )
{
char x[ ] = “MORE JOURNALS” ;
int i = 0 ;
while ( i <= 16 )
{
printf ( “%c”, X[i] ) ;
i++ ;
}
}
Output of the above mentioned program is :
MORE JOURNALS
The %s Format Specifier:
Special character % used in printf() is a format for printing a string. Below mentioned is a program:
#include<stdio.h>
void main( )
{
char name[30] ;
printf ( “Please enter your name ” ) ;
scanf ( “%s”, name ) ;
printf ( “My name is %s!”, name ) ;
}
Output for the program will be:
Enter your name MORE JOURNALS
My name is MORE JOURNALS
The scanf( ):
Two precautions to be taken upon the entering string using scanf() are as mentioned below:
(a) Length of entered stings should be exceeding the dimensions of character array. Because the compiler will not considering characters beyond the limits and because of which something important will be overwritten or missed.
(b) Scanf cannot read multi word strings. To overcome this barrier there is one more function called as gets.
#include<stdio.h>
void main( )
{
char x[25] ;
printf ( “Enter your Blog’s name ” ) ;
gets ( x ) ;
puts ( X ) ;
puts ( “is really very informative ” ) ;
}
And here is the output…
Enter your Blog’s name MORE JOURNALS
MORE JOURNALS
Is really very informative.
Standard Library String Functions in C:
Function | Use |
Strlen | To determine length of a string |
Strlwr | Converts a string to Lower Case |
Strupr | Converts a string to Upper Case |
Strcat | Appends one string at the end of another. |
strncat | Appends first ‘n’ characters of a string at the end of another string. |
strcpy | Copies a string into another string. |
strncpy | Copies first ‘n’ characters of one string into another. |
strcmp | Comparison two strings. |
strncmp | Comparison first ‘n’ characters of two strings. |
strcmpi | Compares two strings without regard to case (“i” denotes that this function ignores case). |
stricmp | Compares two strings without regard to case (identical to strcmpi). |
strnicmp | Compares first ‘n’ characters of two strings without regard to case. |
strdup | Duplicates a string. |
strchr | Finds first occurrence of a given character in a string. |
strrchr | Finds last occurrence of a given character in a string. |
strstr | Finds first occurrence of a given string in another string. |
strset | Sets all characters of string to a given character. |
strnset | Sets first ‘n’ characters of string to a given character. |
strrev | Reverses string. |
What is strlen( ) in C:
A function to count the number of characters present in a strings. A program is written to explain the usage.
#include<stdio.h>
#include<string.h>
voidmain( )
{
char arr[ ] = “MORE JOURNALS ” ;
int len1,
len1 = strlen ( arr ) ;
printf ( “\nArray Length= %s length = %d”, arr, len1 ) ;
}
The output would be…
Array = MORE JOURNALS = 13
What is strcpy( ) function in C:
Content of one string is copied to another using strcpy function. Base address of source and destination string should be given to this function. Below mentioned is the program explaining copy functionality:
#include<stdio.h>
#include<string.h>
voidmain( )
{
char origin[ ] = “MORE JOURNALS ” ;
char destination[20] ;
strcpy ( desitination, origin ) ;
printf ( “\Origin String = %s”, origin ) ;
printf ( “\nDestination string = %s”, destination ) ;
}
Outcome of the above mentioned program is as mentioned below:
Origin string = MORE JOURNALS
Destination string = MORE JOURNALS
Function to concatenate a string:
String at origin is concatenated at the end of the target string. For example “MORE” and “JOURNALS” on concatenation is shown as “MORE JOURNALS ”. A program is written below explaining concatenation of string:
#include<stdio.h>
#include<string.h>
voidmain( )
{
char origin[ ] = “MORE” ;
char destination[30] = “JOURNALS” ;
strcat ( destination,origin ) ;
printf ( “\nOrigin string = %s”, origin ) ;
printf ( “\nDestination string = %s”, destination ) ;
}
Outcome of the above mentioned program is as mentioned below:
Origin string = MORE
Destination string = JOURNALS
Function of comparison of two stings in C:
Comparison of two sting to find out if the two strings are same or different strcmp function is used. Two strings are compared character by character until at least one character mismatches or till the end of the array whichever is occurred. If two strings are identical the value zero is returned. If strings are not identical then numeric difference between the ACII values of the first non matching pairs of character is shown.
/* Program to perform string comparison*/
#include<stdio.h>
#include<string.h>
void main( )
{
char string1[ ] = “Jam” ;
char string2[ ] = “Fan” ;
int i, j ;
i = strcmp ( string1, “Jam” ) ;
j = strcmp ( string1, string2 ) ;
printf ( “\n%d %d %d”, i, j, ) ;
}
Out come of the above mentioned program is as mentioned below:
0 4
For variable I we are storing the return value of two identical string comparison, the comparison was between “Jam” and “Jam” therefore the returned value is zero. In variable J we are storing the value for comparison of two unidentical strings “Jam” and “Fan” and the return values the numeric difference between ASCII value of “J” and ASCII vale of “F”. However difference of ASCII values is not important for us but more relevant is the result of the comparison.
Structure (Grouping of data type together in C):
Grouping together of number of data types is contained in structure. Data type might or might not be of same type. Below mentioned is program:
#include<stdio.h>
viod main( )
{
struct collection
{
char name ;
float salary ;
int age ;
} ;
struct collection b1, b2;
printf ( “\nEnter names, salary & age of 2 employees\n” ) ;
scanf ( “%c %f %d”, &b1.name, &b1.salary, &b1.age ) ;
scanf ( “%c %f %d”, &b2.name, &b2.salary, &b2.age ) ;
printf ( “\nAnd this is what you entered” ) ;
printf ( “\n%c %f %d”, b1.name, b1.salary, b1.age ) ;
printf ( “\n%c %f %d”, b2.name, b2.salary, b2.age ) ;
}
And here is the output…
Enter names, prices and no. of pages of 3 books
A 100.00 354
C 256.50 682
F 233.70 512
Declaring a Structure in C programming:
Below mentioned is an example of defining structure:
struct emp
{
char name ;
float salary ;
int age ;
} ;
Every variable of data type will consist of a character called name, a float variable called as salary and an integer variable called as age. General structure form is mentioned below:
struct <structure name>
{
structure element 1 ;
structure element 2 ;
structure element 3 ;
……
……
} ;
When new structure data type is defined one or more variables can be declared to be of that type. For e.g.
struct emp b1, b2, b3 ;
Statement mentioned above will be having space in memory. It makes available space to hold all the elements in the structure—in this case, 7 bytes—one for name, four for salary and two for age. These bytes are always in adjacent memory locations.