In this post, I will describe about Pointer Notation, Recursion, Recursive Function Program to Calculate Factorial, Integers, long and short, Integers, signed and unsigned, Chars, signed and unsigned, Floats and Doubles and Register Storage Class.
Pointer Notation:
We are declaring the variable, int x = 10 ; to compiler declared variable means:
(a) Memory space is reserved to keep declared integer.
(b) Assign name as i to the memory location
(c) To the stored location save value 3.
For better understanding refer below mentioned:
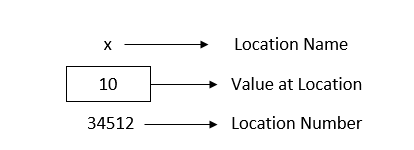
Value 10 is saved in location number 34512 having name as x. Value 10 might not be saved in the same memory location, next time save value will be stored in some other location. The important point is, x’s address in memory is a number. Address number of location name can be printed using a program which is mentioned below:
main( )
{
int x = 10;
printf ( “\nAddress of variable x = %u”, &i ) ;
printf ( “\n Value of x = %d”, i ) ;
}
Output of program will be :
Address of variable x = 34512
Value of x = 10
Recursion:
IF a function calls itself then that function is called as ‘recursive’. If a line of code which is within the body of function calls the same function then that is called as circular definition. Recursion function can be best explained when a program is written to find factorial. Factorial of a number means the multiplication of all the number between that number and 1. For example the factorial of a number is the product of all the integers between 1 and that number. For example, factorial of 5 is 5* 4 * 3 * 2 * 1. Program is written below using non recursive function:
main( )
{
int x, f ;
printf ( “\nPlease enter number for which factorial to be determined:” ) ;
scanf ( “%d”, &x ) ;
f = factorial ( x ) ;
printf ( “Factorial for the entered value is: %d”, f ) ;
}
factorial ( int y )
{
int z = 1, i ;
for ( i = y; i >= 1 ; i– )
z= z * i ;
return ( z ) ;
}
And here is the output…
Please enter number for which factorial to be determined: 5
Factorial for the entered value is: 120
Recursive Function Program to Calculate Factorial:
Program using recursive function is mentioned below:
main( )
{
int x, f ;
printf ( “\nPlease enter number for which factorial is to be determined: ” ) ;
scanf ( “%d”, &x ) ;
f = recursive (x ) ;
printf ( “Factorial for the entered value is: %d”, f ) ;
}
recursive ( int y )
{
int z ;
if ( y == 1 )
return ( 1 ) ;
else
z = y * recursive (y – 1 ) ;
return ( z ) ;
}
And here is the output for four runs of the program:
Please enter number for which factorial is to be determined: 5
Factorial for the entered value is: 120
Integers, long and short:
Compiler decides the range of integer constant. –32768 to 32767 is the range for 16-bit compiler like Turbo C or Turbo C++ and for 32 bit compiler the range is –2147483648 to +2147483647. 16-bit compiler will compiles a C program and it generates machine language that is targeted towards working on a 16-bit microprocessor and 32-bit compiler machine language that is targeted towards a 32-bit microprocessor like Intel Pentium. But this does mean that program compiled using 16 bit compiler will not work with 32 bit processor only difference it will be of time and the efficiency of 32 bit processor will be reduce to 16 bit processor. This happens because a 32-bit processor provides support for programs compiled using 16-bit compilers. But a program compiled using 32 bit process will not work on 16 bit compiler.
Note that two/four bytes used to store an integer, the highest bit (16th /32nd bit) is used to store the sign of the integer. This bit is 1 if the number is negative and 0 if the number is positive.
There are different type of integer data type which are called as short and long integer values. Only purpose having different type is to provide wider range. Compiler itself decides the appropriate size depending upon the OS and hardware in use. Below mentioned are the rules.
(a) shorts are minimum 2 bytes big
(b) longs are at minimum bytes big
(c) shorts are not bigger than ints
(d) ints are not bigger than longs
Sizes of different integers based upon the OS are mentioned below:

Integers, signed and unsigned:
In a situation where we need to perform counting then in that case we know that values will always be positive. Therefore for such vase we can declare a variable to unsigned as mentioned below:
unsigned int Count ;
By using such declaration the range of integer values for 16 bit processor will be increased from -32768 to +32767 to the range 0 to 65535. Therefore if we do such declaration like integer as unsigned then it doubles the possible that can be saved. This happens because upon declaring an integer as unsigned the left most bit will be free and will be used to store the sign of the number. Unsigned integer still occupies two bytes. Unsigned integer can be declared as mentioned below:
unsigned int x ;
unsigned x ;
There is also short unsigned int and long unsigned int but by default short int is signed shot and a long int is signed long int.
Chars, signed and unsigned:
Just like to signed and unsigned ints (either short or long), exist signed and unsigned chars are also available, both occupying one byte. Signed and unsigned chars have different range. One might think that how char can have sign for which please refer below.
char ch = ‘A’ ;
In variable ch binary equivalent ASCII value of character A which is 65 will be store.
Signed char ranges from -128 to 127 and unsigned char ranges from 0 to 255. Now we write a program the tells about the range of char.
#include<stdio.h>
void main( )
{
char x = 291 ;
printf ( “\n%d %c”, x, x ) ;
}
Floats and Doubles:
Float takes 4 bytes and ranges from -3.4e38 to +3.4e38. Also there is double data type which takes 8 bytes and ranges from -1.7e308 to +1.7e308. A variable of type double can be declared as mentioned below:
Double x, numberofstars ;
In certain circumstance real numbers are used for which even double data type is insufficient then for variable to store real number we need to use long double which ranges from -1.7e4932 to +1.7e4932 and takes 10 bytes.
Data Types in C Language:
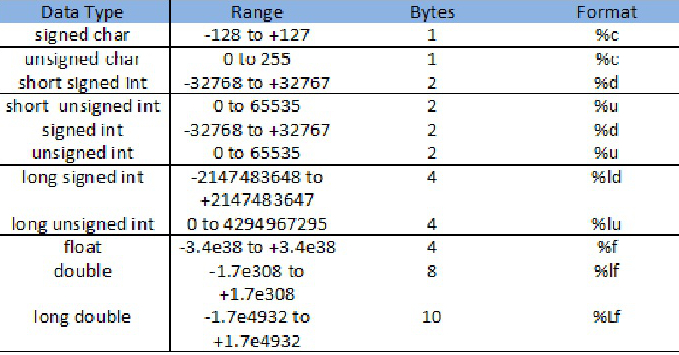
Register Storage Class:
Features of a variable defined to be of register storage class are as under:
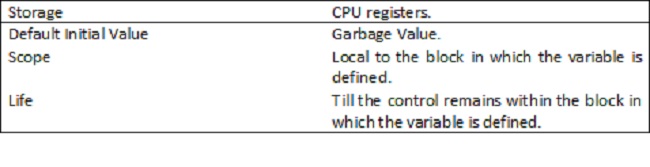
If a variable is used in many places in a program then storage should be declared in register because value saved in CPU register will accessed faster than the one stored in memory. Variable used in loop are the example. Program to save variable in register is a mentioned below:
#include<stdio.h>
void main( )
{
register int x ;
for ( x = 1 ; x <= 10 ; x++ )
printf ( “\n%d”, x ) ;
}