In this post, I will describe about One’s Complement Operator in C programming, Right Shift Operator in C programming, Bitwise AND Operator, Bitwise OR Operator, What is Bitwise XOR Operator in C programming, and showbits( ) Function in C programming.
One’s Complement Operator in C programming:
One’s compliment mean that all the 1’s present in number will be changed to zero and all zero will be changed to 1. For e.g. one’s compliment of 1100110 will be 0011001. Number are should be binary equivalent number. One compliment of 65 means one compliment of 0000 0000 0100 0001, which is binary equivalent of 65. One’s complement of 65 therefore would be, 1111 1111 1011 1110. One’s complement operator is represented by the symbol ~(tilde). Below mentioned is a program explaining about one’s compliment:
#include<stdio.h>
voidmain( )
{
for ( int x = 0 ; x <= 3 ; x++ )
{
printf ( “\nDecimal %d is same as binary “, j ) ;
showbits ( x ) ;
int y = ~x ;
printf ( “\nOne’s complement of %d is “, j )
showbits (y ) ;
}
}
Outcome of above mentioned program is:
Decimal 0 is same as binary 0000000000000000
One’s complement of 0 is 1111111111111111
Decimal 1 is same as binary 0000000000000001
One’s complement of 1 is 1111111111111110
Decimal 2 is same as binary 0000000000000010
One’s complement of 2 is 1111111111111101
Right Shift Operator in C programming:
Use of Right Shift operator is that it shifts each bit to its left operant to the right. Number of place the bits are shifted depend on the number of following the operator. Right Shift operator is shown by >>.
For e.g. ch >>10 would shift all bits in ch ten places to the right. Similarly, ch >> 2 would shift all bits 2 places to the right.
For example, if the variable ch contains the bit pattern 11010111, then, ch >> 1 would give 01101011 and ch >> 2 would give 00110101.
Bits are shifted to the right, blanks are created on the left. These blanks are filled with zero. Below mentioned is a program to explain the use of right shift operator.
#include<stdio.h>
void showbits (int);
void main( )
{
int i = 5225, k ;
printf ( “\nDecimal %d is same as binary “, i ) ;
showbits ( i ) ;
for (int j = 0 ; j <=2 ; j++ )
{
k = i >>j ;
printf ( “\n%d right shift %d gives “, i, j ) ;
showbits ( k ) ;
}
}
The output of the above program would be…
Decimal 5225 is same as binary 0001010001101001
5225 right shift 0 gives 0001010001101001
5225 right shift 1 gives 0000101000110100
127. Left Shift Operator in C programming.
Left shift operator is similar to Right shift operator, but the difference is that the bits re shifted to the left and each bit shift 0 is filled and added to the right of the number.
Below mentioned is program explaining the implementation:
#include<stdio.h>
void showbits (int);
void main( )
{
int x= 5225, y, z ;
printf ( “\nDecimal %d is same as “, x ) ;
showbits ( i ) ;
for ( y = 0 ; y <= 3 ;y++ )
{
z = x <<y;
printf ( “\n%d left shift %d gives “, x, y ) ;
showbits ( z ) ;
}
}
The output of the above program would be…
Decimal 5225 is same as binary 0001010001101001
5225 left shift 0 gives 0001010001101001
5225 left shift 1 gives 0010100011010010
5225 left shift 2 gives 0101000110100100
Bitwise AND Operator:
Bitwise operator is represented as &. It is different that is &&, logical AND operator. & operatory operates on two operands. Operating upon these two operands they are compared on bit by bit basis. Therefor both the operands must be of same type. AND mask is the name given for second operand. & operates on a pair of bits to yield a resultant bit. Rules which decide the value of the resultant bit are shown below:
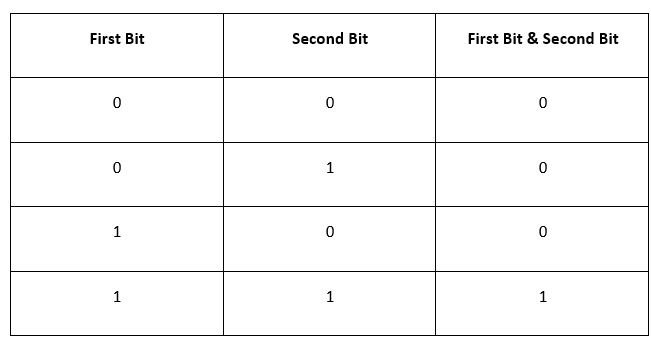
Bitwise OR Operator:
OR operator is important bitwise operator is the OR operator and is represented as |. The rules that govern the value of the resulting bit obtained after ORing of two bits is shown in the truth table below.
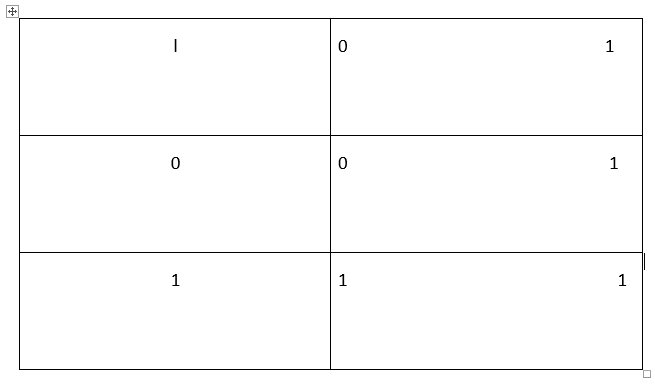
Using the Truth table confirm the result obtained on ORing the two operands as shown below.
11010000 Original bit pattern
00000111 OR mask
————-
11010111 Resulting bit pattern.
Bitwise OR operator is usually used to put ON a particular bit in a number.
Let us consider the bit pattern 11000011. If we want to put ON bit number 3, then the OR mask to be used would be 00001000. All the other bits in the mask are set to 0 and only the bit, which we want to set ON in the resulting value is set to 1.
What is Bitwise XOR Operator in C programming:
Operator XOR is represented as ^ and called as Exclusive OR Operator. OR operator returns 1, wheren any one of two bits or both bits are 1 whereas XOR returns 1 only if one of the two bits is 1. The truth table for the XOR operator is given below:
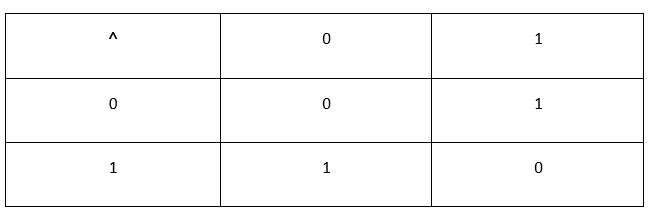
XOR operator is used to toggle a bit ON or OFF. A number XORed with another number twice gives the original number. Below mentioned is program explaining about bitwise XOR operator:
#include<stdio.h>
void main( )
{
int b = 50 ;
b = b ^ 12 ;
printf ( “\n%d”, b ) ; /* this will print 62 */
b = b ^ 12 ;
printf ( “\n%d”, b ) ; /* this will print 50 */
}
The showbits( ) Function in C programming:
Below mentioned is a programming about how showbits function works:
showbits ( int a )
{
int x, y,mask ;
for ( x = 15 ; x >= 0 ;x– )
{
mask = 1 << x ;
y = a & dmask ;
y == 0 ? printf ( “0” ) : printf ( “1” ) ;
}
}
All that is being done in this function is using an AND operator and a variable mask we are checking the status of individual bits. If the bit is OFF we print a 0 otherwise we print a 1.