In this post, I will discuss about Low Level Disk I/O in C Programming, Low level disk I/O function has the below mentioned advantages, Using argc and argv, Standard I / O Devices in C programming, What is I/O functions Redirection and Bitwise Operators in C Programming.
Low Level Disk I/O in C Programming:
Using low level disk I/O data cannot be written as individual characters. Only one way by which data can be written or read in low level disk I/O functions is by buffer full of bytes.
Writing a buffer full of data resembles the fwrite( ) function. But, unlike fwrite( ), the programmer must set up the buffer for the data, place the appropriate values in it before writing, and take them out after writing. Thus, the buffer in the low level I/O functions is very much a part of the program, rather than being invisible as in high level disk I/O functions.
Low level disk I/O function has the below mentioned advantages:
(a) These functions parallel the methods that the OS uses to write to the disk, they are more efficient than the high level disk I/O functions.
(b) Since there are fewer layers of routines to go through, low level I/O functions operate faster than their high level counterparts.
Opening a File:
Before performing any operation on a file at a high level all the files must be opened before accessing.
inhandle = open ( source, O_RDONLY | O_BINARY ) ;
File opened so that communication with operation system regarding file can be established. We need to write open(), file name and mode in which file should be opened. The possible file opening modes are given below:
O_APPEND Opens a file for appending
O_CREAT Creates a new file for writing (has no effect
if file already exists)
O_RDONLY Creates a new file for reading only
O_RDWR Creates a file for both reading and writing
O_WRONLY Creates a file for writing only
O_BINARY Creates a file in binary mode
O_TEXT Creates a file in text mode
Header file for above mentioned functions (O-flags) is“fcntl.h” therefore that file must be included in the program while using low level disk I/O. File “stdio.h” is not necessary for low level disk I/O. When two or more O-flags are used together, they are combined using the bitwise OR operator ( | ).
Other statements that can be used in program to open the file is mentioned below:
outhandle = open ( target, O_CREAT | O_BINARY | O_WRONL, S_IWRITE ) ;
If the target file is not existing then that should be opened we will be using O_CREAT flag, and since file is to be written and not read therefore we have used O_WRONLY. And finally, since we want to open the file in binary mode we have used O_BINARY.
Whenever O_CREAT flag is used, another argument must be added to open( ) function to indicate the read/write status of the file to be created. This argument is called ‘permission argument’.
Permission arguments could be any of the following:
S_IWRITE Writing to the file permitted
S_IREAD Reading from the file permitted
To use these permissions, both the files “types.h” and “stat.h” must be #included in the program alongwith “fcntl.h”.
Using argc and argv:
#include <stdio.h>
#include<stdlib.h>
main ( int argc, char *argv[ ] )
{
FILE *fs, *ft ;
char ch ;
if ( argc != 3 )
{
puts ( “Improper number of arguments” ) ;
exit( ) ;
}
fs = fopen ( argv[1], “r” ) ;
if ( fs == NULL )
{
puts ( “Cannot open source file” ) ;
exit( ) ;
}
ft = fopen ( argv[2], “w” ) ;
if ( ft == NULL )
{
puts ( “Cannot open target file” ) ;
fclose ( fs ) ;
exit( ) ;
}
while ( 1 )
{
ch = fgetc ( fs ) ;
if ( ch == EOF )
break ;
else
fputc ( ch, ft ) ;
}
fclose ( fs ) ;
fclose ( ft ) ;
}
Standard I / O Devices in C programming:
Fopen() function is used to perform reading or writing operations in which function sets up a file pointer to refer the file. Many operating system pre-defines pointers for three standard files. To access these pointers its no necessary to user fopen().
Standard File Pointer Description
Stdin Standard input device (Keyboard).
Stdout Standard output device (VDU).
Stdderr Standard error device (VDU).
Ch=fgetc(stdin) will read a character from keyboard rather than from a file because of this we don’t need use f.open or f.close functions,
What is I/O functions Redirection:
Powerful features allowing a program to read or write files are incorporate in operating system even if such functionalities are not incorporated in the program. This is done through a process called ‘redirection’.
Keyboard and VDU are the input and output devices in C program. This means that there are some assumptions being made like from where input is coming and to where output should do. Redirection allows us to change these assumptions.
Using redirection the output of program can be changed from VDU to printer or to disk without using a program. This approach is more convenient and flexible than providing separate function.
To use redirection facility is to execute the program from the command prompt, inserting the redirection symbols at appropriate places.
Bitwise Operators in C Programming:
Using program one can perform manipulation of bits by bit manipulation operators. With bit manipulation operation individual bits can be manipulated within a piece of data. Below mentioned are Bitwise operator used in C programming:
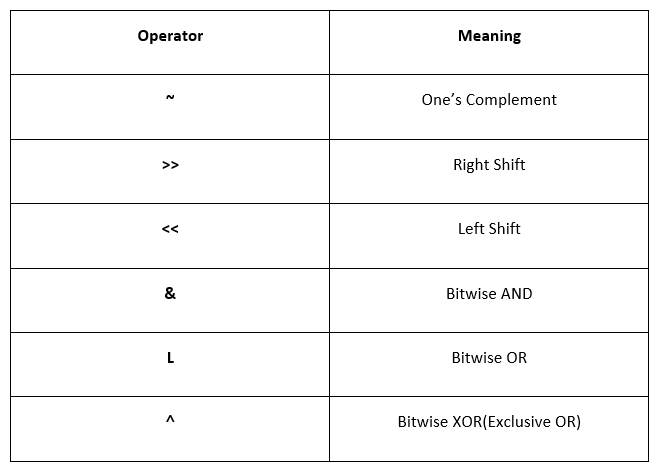
These operators can perform upon ints and chars but not on float and doubles.
These operators can operate upon ints and chars but not on floats and doubles. Before moving on to the details of the operators, Bits are numbered from zero onwards, increasing from right to left as shown:
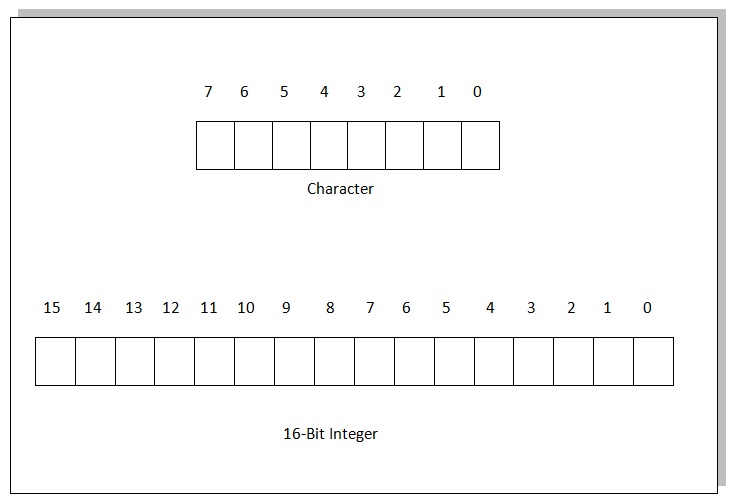