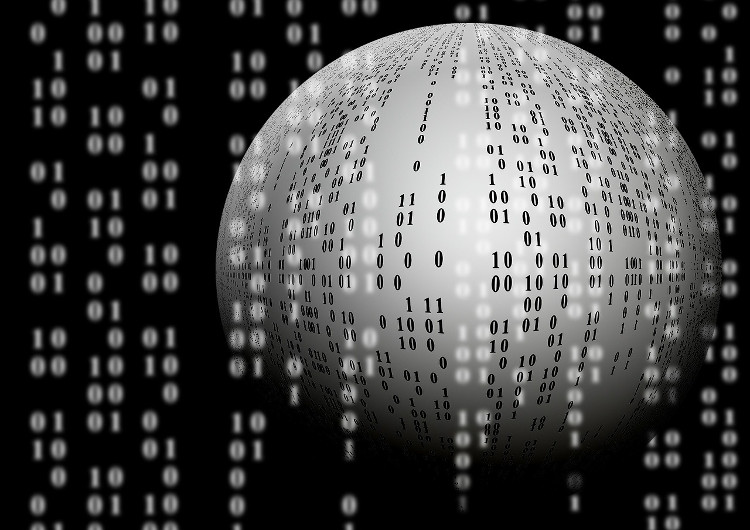
In this post, I will discuss about Writing to a File in C using a function, File Opening Modes in C, String (line) I / O in Files: in C programming, Record Input-Output Files using C program, Text Files and Binary Files in C programming, Text versus Binary Mode files- Newlines in C programming, End of File read in Text Mode and Binary mode files and Time versus Binary Mode- Storage of Numbers.
Writing to a File in C using a function:
Function fputc() is similar to putch() because for both output result are characters. However putch() function writes to VDU, where fputc() writes in the files. File to written is signified by ft. Writing is continuous until all characters from source file have been written to destination file.
File Opening Modes in C:
There are many modes in which a file can be opened like r mode where file can be read but no append can be performed. Below mentioned are the different file opening modes. The tasks performed by fopen( ) when a file is opened in each of these modes are also mentioned.
“r” | Finds the file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file cannot be opened fopen( ) returns NULL. Opened file is in the read only mode. |
“w” | Finds the file. If the file exists, its contents are overwritten. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file. File will be opened in editable mode. |
“a” | Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer that points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file. Operations possible – adding new contents at the end of file. |
“r+” | Searches file. If is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. Returns NULL, if unable to open the file. Operations possible – reading existing contents, writing new contents, modifying existing contents of the file. |
“w+” | Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist a new file is created. Returns NULL, if unable to open file. Operations possible – writing new contents, reading them back and modifying existing contents of the file. |
“a+” | Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file. Operations possible – reading existing contents, appending new contents to end of file. Cannot modify existing contents. |
String (line) I / O in Files: in C programming
In some situations the usage of I/O functions that read or write entire strings might turn out to be more efficient. Reading or writing strings of characters from and to files is as easy as reading and writing individual characters. Below is a program that writes string to a file using fputs() function:
/* A Program to receive strings from keyboard and writes them to file */
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void main( )
{
FILE *fp ;
char string[80] ;
fp = fopen ( “MoreJournals.TXT”, “w” ) ;
if ( fp == NULL )
{
puts ( “MoreJournals file cannot be opened” ) ;
exit(1 ) ;
}
printf ( “\n Please enter a few lines of text:\n” ) ;
while ( strlen ( gets ( string ) ) > 0 )
{
fputs ( string, fp ) ;
fputs ( “\n”, fp ) ;
}
fclose ( fp ) ;
}
Output for the above written program is as mentioned below:
In MoreJournals.com you will get technology related articles.
Record Input-Output Files using C program:
In this article we will be discussing on how to read or writes numbers from a file or to a file respectively. For reading or writing first we have organize the dissimilar data together in a structure and then use fprint() and fscanf() function to read/write from/to file.
/* Writes records to a file using structure */
#include <stdio.h>
#include<conio.h>
void main( )
{
FILE *fp ;
char another = ‘T’ ;
struct emp
{
char name[40] ;
int age ;
float bs ;
} ;
struct emp e1 ;
fp = fopen ( “EMPLOYEE.DAT”, “w” ) ;
if ( fp == NULL )
{
puts ( ” Sorry file cannot be opened” ) ;
exit( ) ;
}
while ( another == ‘T’ )
{
printf ( “\nEnter name, age and basic salary of employee: ” ) ;
scanf ( “%s %d %f”, e1.name, &e1.age, &e1.bs ) ;
fprintf ( fp, “%s %d %f\n”, e1.name, e1.age, e1.bs ) ;
printf ( “Add another record (Y/N) ” ) ;
fflush ( stdin ) ;
another = getche( ) ;
}
fclose ( fp ) ;
}
And here is the output of the program…
Enter name, age and basic salary: Sunil 30 600000
Add another record (Y/N) N
Text Files and Binary Files in C programming:
A binary file is collection of bytes. This collection could be a compiled version of program. Easiest way to find out if a file is text or binary file is the open that file in Turbo C/C++. IF on open a the file you can interpret what is displayed then it is a text file else it is a binary file.
Below is a program that is capable of copying text as well as binary files as shown below.
#include <stdio.h>
#include<stdlib.h>
voidmain( )
{
FILE *fs, *ft ;
int ch ;
fs = fopen ( “pr1.exe”, “rb” ) ;
if ( fs == NULL )
{
puts ( “Source file cannot be opened” ) ;
exit(1 ) ;
}
ft = fopen ( “newpr1.exe”, “wb” ) ;
if ( ft == NULL )
{
puts ( “Target file cannot be opened” ) ;
fclose ( fs ) ;
exit(2 ) ;
}
while ( 1 )
{
ch = fgetc ( fs ) ;
if ( ch == EOF )
break ;
else
fputc ( ch, ft ) ;
}
fclose ( fs ) ;
fclose ( ft ) ;
}
Source and target files are opened in “rb” and “wb” modes respectively. While opening the file in text mode we can use either “r” or “rt”, but since text mode is the default mode usually ‘t’ is not mentioned.
Text versus Binary Mode files- Newlines in C programming:
In text mode a new line character is converted into carriage returnline feed combination before being written to the disk. And also carriage return line feed combination on disk is converted to newline when file is read by C program, but if the file is opened in binary mode as opposed to text mode then these conversions will be not be performed.
End of File read in Text Mode and Binary mode files:
In text mode a special character whose ASCII value is 26 is inserted after the last character of the file which means that it is the end of file. IF this character is detected at any point in the file, then read function will return the End of file. But in binary mode files there is no such special character present. Binary mode files keep track of end of file from the number of character present in directory entry of the file.
Binary mode only be used for reading numbers back, there might be a possibility that number stored can be 26 (hexadecimal 1A). If the same file is opened in text mode for reading then the reading will be terminated.
Thus the two modes are not compatible. There is it important that the file that has been written in text mode is read back only in text mode. Similarly, the file that has been written in binary mode must be read back only in binary mode.
Time versus Binary Mode- Storage of Numbers:
Fprintf() is the only function which is available for storing numbers in a disk file. It is very important to under the concept of how numerical data is stored on the disk by fprintf() function. Characters and strings are stored one character per byte, number stored as they are in memory, two bytes for integer, 4 bytes for float.
Number are stored as strings of characters. Thus 1234 even though it should be occupying two bytes in memory but when transferred to disk using fprintf(), it would occupy 4 bytes, one byter per characters. Number with more digits would require more space.
There if large amount of numerical data is to be stored in a disk file, using text mode may turn out to be inefficient. The solution is to open the file in binary mode and use those functions like fread and fwrite which store the numbers in binary format. It means each number would occupy same number of bytes on disk as it occupies in memory.