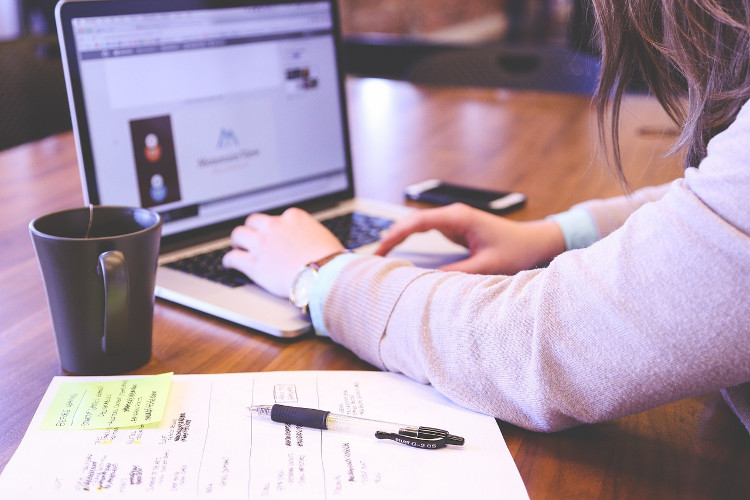
In this post I will describe Cyclomatic complexity in Software Testing and how to calculate code complexity using Cyclomatic complexity. I have included few examples to understand how the code complexity is calculated. Also, I have mentioned advantages of using Cyclomatic Complexity.
Definition: Cyclomatic complexity (or conditional complexity) is a measurement or a software metric. Cyclomatic complexity was developed by Thomas J. McCabe, Sr. in 1976 and is used to calculate the complexity of a program or a code. It directly measures the number of linearly independent paths through a program’s source code. The concept, although not the method, is somewhat similar to that of general text complexity measured by the Flesch-Kincaid Readability Test.
Cyclomatic complexity is computed using the control flow graph of the program: the nodes of the graph correspond to indivisible groups of commands of a program, and a directed edge connects two nodes if the second command might be executed immediately after the first command. Cyclomatic complexity may also be applied to individual functions, modules, methods or classes within a program.
One testing strategy, called Basis Path Testing by McCabe who first proposed it, is to test each linearly independent path through the program; in this case, the number of test cases will equal the cyclomatic complexity of the program.
Cyclomatic complexity is a computer science metric (measurement) developed by Thomas McCabe used to generally measure the complexity of a program. It directly measures the number of linearly independent paths through a program’s source code.
The concept, although not the method, is somewhat similar to that of general text complexity measured by the Flesch-Kincaid Readability Test.
Cyclomatic complexity is computed using a graph that describes the control flow of the program. The nodes of the graph correspond to the commands of a program. A directed edge connects two nodes, if the second command might be executed immediately after the first command. By definition,
CC = E – N + P
where
CC = cyclomatic complexity
E = the number of edges of the graph
N = the number of nodes of the graph
P = the number of connected components
Cyclomatic complexity defines, how much complex does our computer program is. means, it defines the overall degree of hardness in working of our program.
If we are working through flow graphs, we can define cyclomatic complexity(CC) as …
CC= P + 1….
i.e.
P- no. of predicate nodes. Which means no. of nodes from which two or more than two edges are falling out.
From a layman’s perspective the above equation can be pretty daunting to comprehend. Fortunately there is a simpler equation which is easier to understand and implement by following the guidelines shown below:
Start with 1 for a straight path through the routine.
Add 1 for each of the following keywords or their equivalent: if, while, repeat, for, and, or.
Add 1 for each case in a switch statement.
Let’s look at a few examples to understand how the code complexity is calculated.
Example 1:
publicvoid ProcessPages()
{
while(nextPage !=true)
{
if((lineCount<=linesPerPage) && (status != Status.Cancelled) && (morePages == true))
{
//….
}
}
In the code above, we start with 1 for the routine, add 1 for the while loop, add 1 for the if, and add 1 for each && for a total calculated complexity of 5.
Example 2:
publicint getValue(int param1)
{
int value = 0;
if (param1 == 0)
{
value = 4;
}
else
{
value = 0;
}
return value;
In the code above, we start with 1 for the routine, add 1 for the if, and add 1 for the else for a total calculated complexity of 3.
Members that have high code complexity should be reviewed for possible refactoring.
Tools:
- DevMetrics by Anticipating minds have a free community edition available for analyzing metrics for C# projects.
- Reflector Add-In: Code Metrics can be used to analyze .NET assemblies and show design quality metrics. This add-in is to be used in conjunction with Lutz Roeder’s Reflector.
Advantages of using Cyclomatic Complexity:
- It is very easy to compute as illustrated in the example.
- Unlike other complex measurements, it can be computed immediately in the development cycle (which makes it agile friendly).
- It provides a good indicator of the ease of code maintenance.
- It can help focus testing efforts.
- It makes it easy to find complex code for formal review.