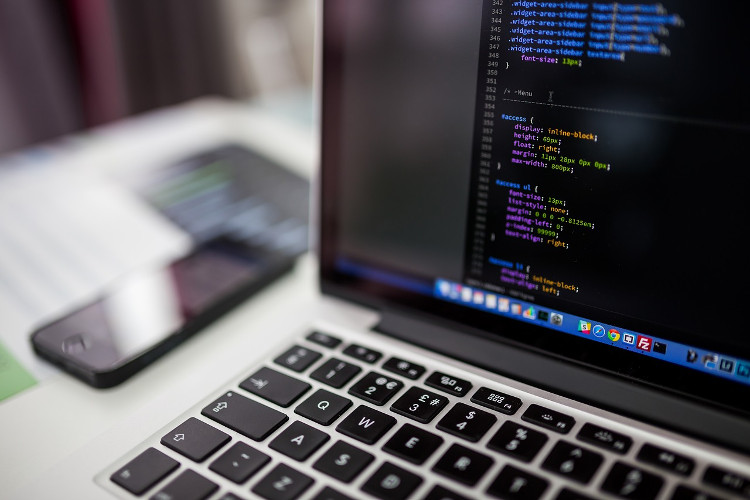
What is Linux?
Linux operating system is clone of Unix operating system. Kernel of Linux was developed from scratch by Linus Torvalds with assistance from a loosely-knit team of programmers across the world on internet. It has got all the features that would be expected in a modern operating system. Unlike windows or Unix, Linux is totally open source.
Kernel of Linux is available in source code form. Anyone is allowed to change the match their requirement, with a pre-condition that the modified kernel can be only distributed only in the source code form. Many programs, frameworks, utilities have been built around linux kernel. They also provide installation scripts for easy installation o linux OS and application.
Few popular distribution scripts for easy installations of linux OS and application. Redhat, SUSE, Caldera, Debian, Mandrake, Slackaware are some of the popular distributions. Each of them contain sam kernel but may be having different application programs, libraries, frameworks, installation scripts, utilities, etc.
Linux was first developed for x86-based PCs (386 or higher). These days it also runs on Compaq Alpha AXP, Sun SPARC, Motorola 68000 machines (like Atari ST and Amiga), MIPS, PowerPC, ARM, Intel Itanium, SuperH, etc. Thus Linux works on literally every conceivable microprocessor architecture.
Under Linux one is faced with simply too many choices of Linux distributions, graphical shells and managers, editors, compilers, linkers, debuggers, etc.
Linux Distribution | Red Hat Linux 9.0 |
Console Shell | BASH |
Graphical Shell | KDE 3.1-10 |
Editor | KWrite |
Compiler | GNU C and C++ compiler (gcc) |
How to do C Programming Under Linux:
C programming in linux can be same and is also different in few ways. It is same to the extent that the language elements like data types, control instructions and syntax. Use of standard library function is same even if the implementation of each might be different under different operating systems. Coders will be not be facing any difference because they will continue to use printf() as they will be using in anyother operating system irrespective of how printf() will be implemented.
But if we will be writing programs that utilize the feature provided by operating system then it will be different programming. For example if we will be writing a C program that will be creating a window or displaying a message then architecture of such programs will be closed related with operating system in which program is written. This is so because mechanism to create window or reporting a mouse click or handling mouse click, displaying message will be different related to operating system. Hence naturally the program that achieves the same task under different OS would have to be different.
Parent and Child Processes:
An executing program is considered as process and this process can create another process. There is parent-child relationship between two processes. The way to achieve this is by using a library function called fork( ). This function splits the running process into two processes, the existing one is known as parent and the new process is known as child. Below is a program explaining :
#include<stdio.h>
# include <sys/types.h>
void main( )
{
printf ( “Before Forking\n” ) ;
fork( ) ;
printf ( “After Forking\n” ) ;
}
Here is the output of the program…
Before Forking
After Forking
After Forking
If we observe the output of the program then we will find that all the statement after fork() are executed twice once the parent process and second time by child process. In other words fork( ) has managed to split our process into two.
Zombies and Orphans process in Linux:
We have an idea that a command lists all the running process but from does the program will be getting information. Linux will be having table containing information about all the processes which is called as “Process Table”. Apart from other information process table will be having entry of ‘exit code’ of process. Integer value indicates the reason why the process was terminated.
Even the process comes to an end its entry would remain process table till the time that parent of the terminated process queries the exit code. This process of querying delete the entry of terminated process from process table and returns the exit code to the parent that raised the query.
When we fork a new child process and the parent and the child continue to execute then there are two possibilities either child process ends first or end after parent process.
If child process terminates before the parent process then in this case till the time parent process does not query the exit code of the terminated child the entry of the child process would continue to exist. Such a process in Linux terminology is known as a ‘Zombie’ process. Zombie means ghost, or in plain simple Hindi a ‘Bhoot’. Moral is, a parent process should query the process table immediately after the child process has been terminated. This would prevent a zombie.
But if the parent terminates without querying then in such a case the zombie child process will be an ‘Orphan’ process. Immediately, the father of all processes—init—adopts the orphaned process. Next, as a responsible parent init queries the process table as a result of which the child process entry is eliminated from the process table.
If parent process is terminated before the child process then since every parent process is launched from the Linux shell, the parent’s parent is the shell process. When the parent process is terminated, then shell queries the process table. Therefore a proper cleanup will be begin for the parent process.
However, the child process which is still running is left orphaned. Then immediately the init process would adopt it and when its execution is over init would query the process table to clean up the entry for the child process. And in this case the child process will not be a zombie.
Therefore when a zombie process or an orphan process gets created the operating system take over and ensures that a proper cleanup of relevant process table entry happens.
It is the important to have cleanup which can be done from our programming practice where program shall the get the exit code of the terminated process. Proper exit code of terminated process is important because it tell if the assigned task to process is successful or not.