In this post, I will discuss Array Initialization, Array Elements in Memory, Bounds Checking, Passing Array Elements to a Function, Passing an Entire Array to a Function, Two Dimensional Array, Array of Pointers, Arrangement of the Array of Pointers in Memory and Three-Dimensional Array in C language.
Array Initialization:
We will be discussing in this article about initialization of an array while we are declaring an array. Below mentioned are few example to array initialization and declaration:
Int week [6] = {Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday}
int number[5] = { 1, 2, 3, 4, 5} ;
float percentage[ ] = { 14.6, 17.2 -22.4, -33.3 } ;
Few important points to be considered while initializing and declaring:
(a) Until the array element are initialized with specific values, they are supposed to contain garbage values.
(b) Mentioning the dimension for an array is option.
Array Elements in Memory:
Consider the following array declaration:
int a[5] ;
When array is declared 16 bytes is reserved in memory, 2 bytes each for 8 integers if window or linux array would occupy 32 bytes each integer would occupy 4 bytes. Since the value is not been initialized, all 5 values will be having garbage values, assigning garbage values takes place automatically. If the storage class is declared to be static then all the array elements should be having a default initial value zero. Irrespective of initial values all the array elements will be present in contiguous memory location. ent in contiguous memory locations. This arrangement of array elements in memory is shown in figure below.
Bounds Checking:
If subscript used for an array is exceeding the size of the array cannot be evaluated. If the entered data with subscript is exceeding the size of the array then data will be place in memory outside the array which will cause unexpected output and also error message or warning regarding array out of memory will not be shown. Example for such a program is mentioned below:
#include<stdio.h>
void main( )
{
int number[10] ;
for (int i = 0 ; i <= 50 ; i++ )
num[i] = i ;
}
Passing Array Elements to a Function:
Functions using call by value or call by reference can be used to passed array elements to a function. In call by value we will be passing the value of array element to the function and in call by reference address of array elements to the function Below mentioned are programs explaining call by reference and call by value:
/* Program to show passing array values using call by value */
#include<stdio.h>
void display (int);
void main( )
{
int week[ ] = { 1, 2, 3, 4, 5, 6,} ;
for ( int i = 0 ; i <= 6 ; i++ )
display ( week[i] ) ;
}
void display ( int x )
{
printf ( “%d “,x ) ;
}
Output of the above written program is
1 2 3 4 5 6
We are passing value of each array elements to function display and getting displayed.
/* Program to show passing array values using call by reference */
#include<stdio.h>
void disp(int *);
void main( )
{
int week[ ] = { 1, 2, 3, 4, 5, 6,} ;
for (int i = 0 ; i <= 6 ; i++ )
display ( &week[i] ) ;
}
void disp ( int *n )
{
printf ( “%d “, *n ) ;
}
Output of above written program is as mentioned below.
1 2 3 4 5 6
We are passing address of elements of array to display. Variable in which this address is collected is declared as pointer variable.
Passing an Entire Array to a Function:
We will be discussing in this article about how we will be passing entire array to a function. Below mentioned is an example:
/*Program to show entire array passing to a function */
#include<stdio.h>
void display(int *, int);
void main( )
{
int week[ ] = { 2, 3, 4, 5, 6, 7 } ;
display ( &num[0], 6 ) ;
}
void display ( int *j, int n )
{
for (int i = 0 ; i <= n – 1 ; i++ )
{
printf ( “\nelement = %d”, *j ) ;
j++ ; /* increment pointer to point to next element */
}
}
Display function is the above program is used to print the array elements declared and initialized in the program. Address of the zeroth element will be passed to display function. For loop works to access toe array elements using pointers. By passing only the zeroth element of array to functions equivalent to passing the complete array to function. It is important to pass the total number of elements in the array else for display will never come to know when to terminate for loop. Zeroth element also called are base address can be passed by passing the name array.
Two Dimensional Array:
Array can be one dimension or two dimension, two dimension array is called as matrix. Below is program an example of two dimensional array.
#include<stdio.h>
void main( )
{
int marks[4][2] ;
int j ;
for ( int i = 1 ; i <= 4 ; i++ )
{
printf ( “\n Enter roll no. and marks” ) ;
scanf ( “%d %d”, &marks[i][0], &marks[i][1] ) ;
}
for ( i = 1 ; i <= 4 ; i++ )
printf ( “\n%d %d”, marks[i][0], marks[i][1] ) ;
}
Array of Pointers:
Array of pointers also exists juts like array of int or an array of float. Collection of addresses will be called as array of pointers because pointers are variables containing addresses. Addresses in an array can be a collection of isolated address or group of addresses. Rules applicable for array of pointers are same as that of ordinary array. For further clarification a program is written below:
#include<stdio.h>
void main( )
{
int *array[3] ; /* array of integer pointers */
int x = 45, y = 79, z = 99,
array[0] = &x;
array[1] = &y;
array[2] = &z;
for ( int a = 0 ; a <= 2 ; a++ )
printf ( “%d “, * ( array[a] ) ) ;
}
Arrangement of the Array of Pointers in Memory:
How values and arrangement of array of pointers in memory is shown below in figure. Array contain addresses of isolated integer variable x, y, z. For loop picks the addresses present in “array” and prints the values allocated to addresses.
Three-Dimensional Array:
After understanding the concept of two dimensional array we will be discussing about three dimensional array, though three dimensional array very rare to use. Below mentioned is an example of initializing a three dimensional array:
int arr[3][4][2] = {
{
{ 13, 64 },
{ 47, 48 },
{ 35, 84 },
{ 85, 36 }
},
{
{ 71, 16 },
{ 73, 34 },
{ 95, 23 },
{ 70, 93 }
},
{
{ 98, 19 },
{ 77, 32 },
{ 6, 10 },
{ 50, 11 },
}
} ;
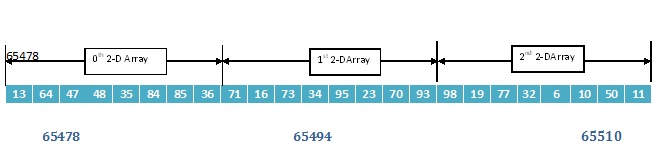
Three dimensional array can be visualized as array of arrays of arrays. The outer array has three elements, each of which contains two integers. In other words, a one-dimensional array of two elements is constructed first. Four one dimensional arrays are placed one below the other to give a two-dimensional array containing four rows. Then, three such two-dimensional arrays are placed one behind the other to yield a three-dimensional array containing three 2-dimensional arrays. In the array declaration note how the commas have been given. Figure below would possibly help you in visualizing the situation better.